In modern application development, user interfaces are pivotal in creating intuitive and interactive experiences. One fundamental UI component used extensively is the radio button. In this article, we will explore the concept of radio buttons in Jetpack Compose, the declarative UI toolkit for Android, and provide practical examples to showcase their implementation. By the end, you’ll clearly understand how to utilize radio buttons effectively in your Compose projects.
Page Contents
Understanding Radio Buttons:
Radio buttons are a UI element that allows users to select a single option from a predefined list of mutually exclusive choices. When one radio button is selected, any previously selected option is automatically deselected. This behaviour ensures that only one choice can be active at a time, making radio buttons ideal for scenarios where exclusive selection is required, such as choosing a gender or a payment method.
Implementing Radio Buttons in Jetpack Compose:
Jetpack Compose simplifies UI development by providing a declarative approach, allowing developers to define UI components as functions that describe their desired state. To create radio buttons in Compose, follow these steps:
Step 1: Import the necessary Compose libraries and dependencies:
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.selection.selectable
import androidx.compose.foundation.selection.selectableGroup
import androidx.compose.material.RadioButton
import androidx.compose.runtime.*
import androidx.compose.ui.Modifier
Step 2: Define the radio button options and their corresponding states:
enum class RadioButtonOptions {
OPTION_1, OPTION_2, OPTION_3
}
@Composable
fun RadioButtonExample() {
var selectedOption by remember { mutableStateOf(RadioButtonOptions.OPTION_1) }
val options = RadioButtonOptions.values()
}
Step 3: Create the radio button group and options:
Column {
options.forEach { option ->
RadioButton(
selected = selectedOption == option,
onClick = { selectedOption = option },
modifier = Modifier.selectable(
selected = selectedOption == option,
onClick = { selectedOption = option }
)
)
}
}
Step 4: Utilize the radio button component:
@Composable
fun RadioButtonsScreen() {
RadioButtonExample()
}
Example Usage:
To demonstrate the usage of radio buttons in Compose, let’s consider an example where we want users to select their favourite colour from a predefined list.
@Composable
fun ColorSelectionScreen() {
var selectedColor by remember { mutableStateOf("") }
val colors = listOf("Red", "Blue", "Green")
Column {
colors.forEach { color ->
RadioButton(
selected = selectedColor == color,
onClick = { selectedColor = color },
modifier = Modifier.selectable(
selected = selectedColor == color,
onClick = { selectedColor = color }
)
)
}
}
}
Conclusion:
Radio buttons are a crucial UI component for implementing exclusive selection options in your applications. With Jetpack Compose, creating and utilizing radio buttons becomes straightforward, thanks to its declarative nature. By following the steps outlined in this article and using the provided example programs, you can easily incorporate radio buttons into your Compose projects, enabling users to make single selections from a list of options with ease and efficiency.
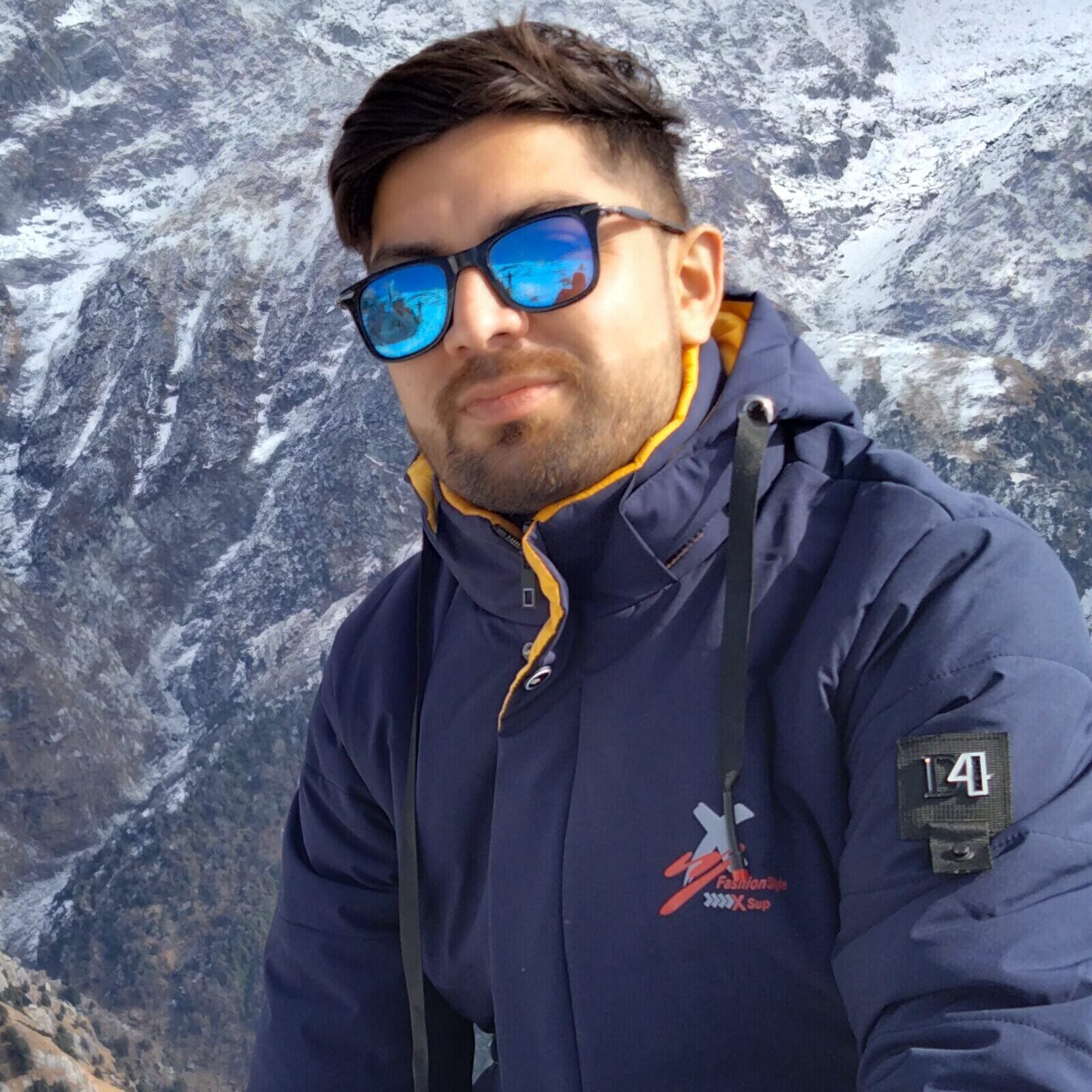
Parvesh Sandila is a passionate web and Mobile app developer from Jalandhar, Punjab, who has over six years of experience. Holding a Master’s degree in Computer Applications (2017), he has also mentored over 100 students in coding. In 2019, Parvesh founded Owlbuddy.com, a platform that provides free, high-quality programming tutorials in languages like Java, Python, Kotlin, PHP, and Android. His mission is to make tech education accessible to all aspiring developers.​