In this tutorial, we will learn about PHP Cookies. PHP Cookies are used to store some data on client’s machines in the form of a text file. The main motive of storing cookies on the client machine is to identify the user when user will revisit a site. Because whenever a user requests a web page to the server it also sends cookies to the server. To understand it you can check the following illustration.
Page Contents
As in the above illustration, you can see the first time the user is just sending a normal request to the server. But the server is giving back response and cookies to the client and after this when the client again try to send a request to the server it is sending cookies back to the server with the request.
Creating PHP cookies:
We have setcookie() function available in PHP to create a cookie. For example, you want to save a cookie to store the name of the user.
Syntax of setcookies():
setcookie(cookie_name, cookie_value, expire_time, path, domain, secure, httponly);
In the above syntax except for cookie_name, all the other parameters are optional. Please check out the following example program.
Example Program:
<?php
$cookie_name = "user_name";
$cookie_value = "Rahul";
setcookie($cookie_name, $cookie_value);
?>
Fetching Cookies variables:
After storing PHP cookies on the client’s machine we will get all the cookies variable and values when user will revisit the site. Here is an example program to show how we can fetch these cookies variables using $_COOKIE global variable.
Example Program:
<?php
$cookie_name = "user_name";
$cookie_value = "Rahul";
setcookie($cookie_name, $cookie_value);
if(isset($_COOKIE[$cookie_name]))
{
echo "Hello ". $_COOKIE[$cookie_name];
}
?>
Updating the value of the cookie variable:
We can update the value of cookie variable any number of time using setcookie() function.
Example Program:
<?php
$cookie_name = "user_name";
$cookie_value = "Rahul";
setcookie($cookie_name, $cookie_value);
?>
Deleting cookie variables:
To delete the cookie we can use setcookie() function with an expiration date in the past.
Example Program:
<?php
$cookie_name = "user_name";
$cookie_value = "Rahul";
setcookie($cookie_name, $cookie_value,time() - 3600);
?>
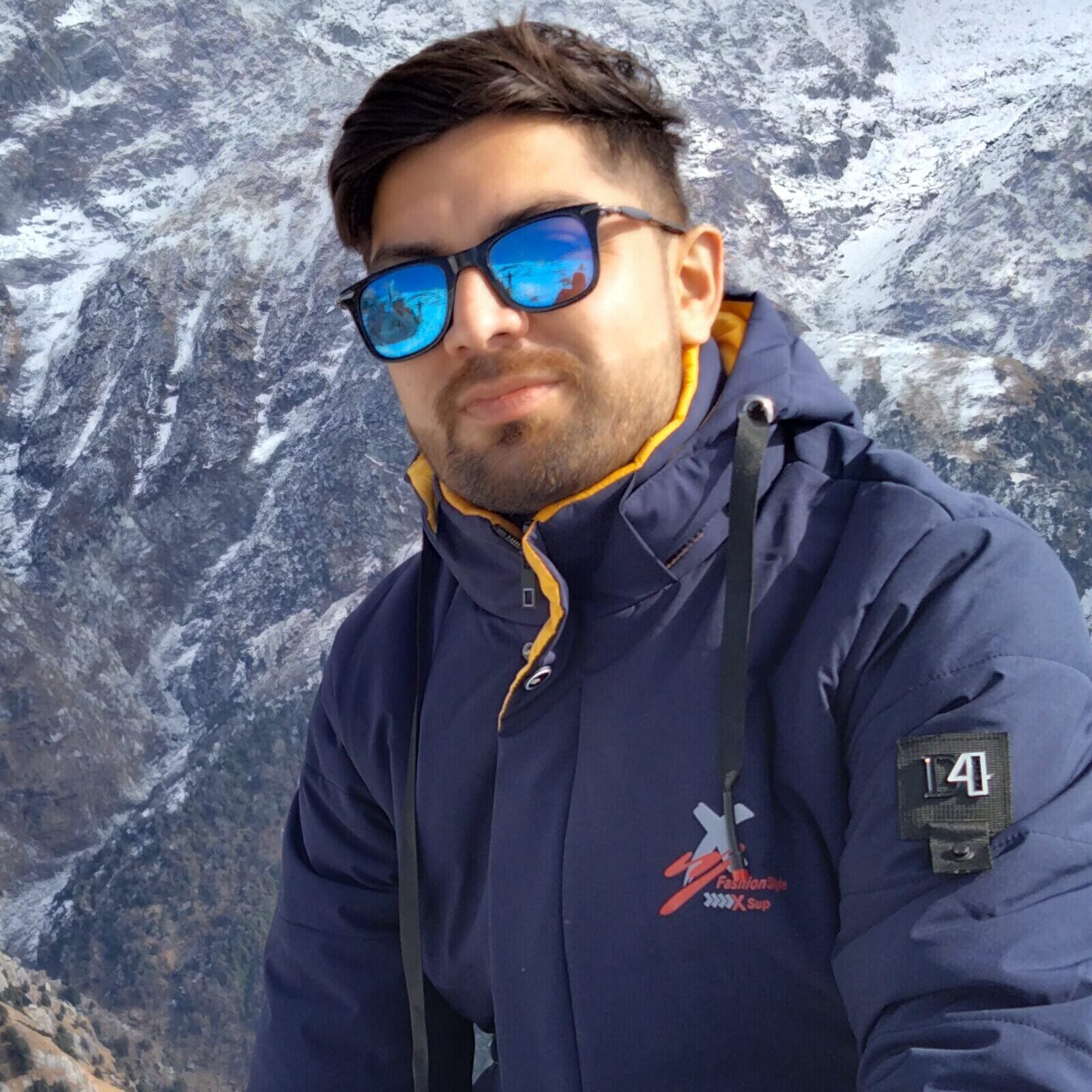
Parvesh Sandila is a passionate web and Mobile app developer from Jalandhar, Punjab, who has over six years of experience. Holding a Master’s degree in Computer Applications (2017), he has also mentored over 100 students in coding. In 2019, Parvesh founded Owlbuddy.com, a platform that provides free, high-quality programming tutorials in languages like Java, Python, Kotlin, PHP, and Android. His mission is to make tech education accessible to all aspiring developers.