In this tutorial, we’ll dive into Inheritance in PHP. It’s a way to inherit properties and methods from one class to another, allowing us to create a new class with its features, plus all the attributes of its parent class. During inheritance, you’ll encounter two types of classes
Page Contents
- Parent Class: The original class that shares its properties and methods.
- Child Class: The class that gains features from another class through inheritance.
Note, extends keyword is used to perform inherit
Available Types of Inheritance in PHP:
- Single Inheritance
- Multilevel Inheritance
- Hierarchical Inheritance
Those who have background in c++ might be missing Multiple Inheritance. But Multiple Inheritance is not available in PHP.
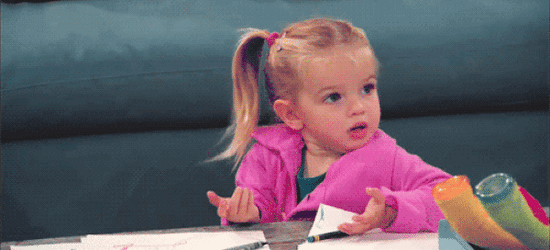
Single Level Inheritance:
In single-level inheritance, one class is inherited into another, with one serving as the parent class and the other as the child class. This straightforward form of inheritance is commonly referred to as simple inheritance.
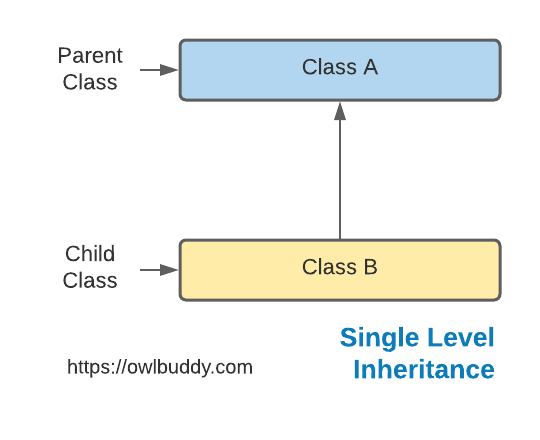
Example Program:
<?php
//Parent Class
class Person {
protected $name;
function set_name($name){
$this->name = $name;
}
}
//child class inheriting Person class
class Student extends Person {
function say_hello(){
echo "Hello $this->name, How is your study going on?";
}
}
$student1 = new Student;
$student1->set_name("Rahul");
$student1->say_hello();
?>
In the example program above, notice that the $name variable and set_name() method are not explicitly written in the Student class. However, we can access them using the object of the Student class. This happens because, after inheriting the Person class in the Student class, the properties and methods seamlessly become part of the Student class.
Multilevel Inheritance:
In this form of inheritance, more than two classes are involved. In Multilevel inheritance, a parent class is inherited by a child class, and then this child class becomes the parent for another child class. This creates a chain of inheritance, extending the features through multiple levels.
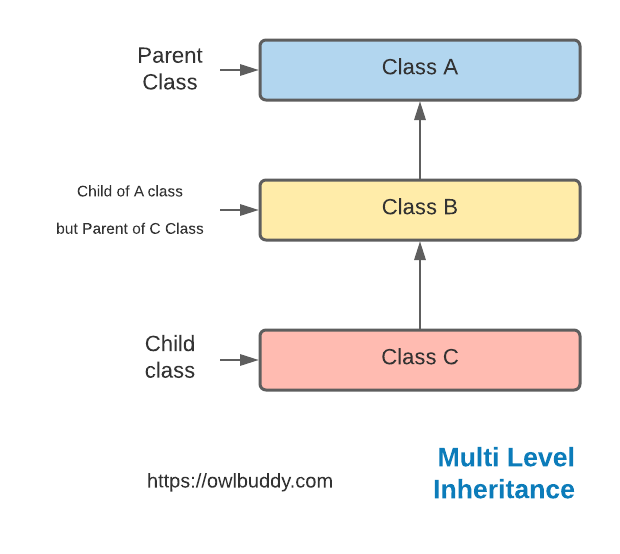
In above scanrio, B is parent of C class and also A is indrect parent of C class.
Example Program:
<?php
class A{
function showA(){
echo "I am show method in A Class<br>";
}
}
class B extends A{
function showB(){
echo "I am show method in B Class<br>";
}
}
class C extends B{
function showC(){
echo "I am show method in C Class<br>";
}
}
$obj=new C;
$obj->showA();
$obj->showB();
$obj->showC();
?>
Hierarchical Inheritance:
In Hierarchical Inheritance, a single class inherits from more than one class. This creates a branching structure where multiple child classes inherit from a common parent class. Check the illustration below to better grasp this concept.

Example Program:
<?php
class Greeting{
function welcome($str){
echo "Hello, We welcome you $str <br>";
}
}
class Teacher extends Greeting{
}
class Student extends Greeting{
}
$teacher = new Teacher;
$teacher->welcome("Sir");
$student =new Student;
$student->welcome("dear");
?>
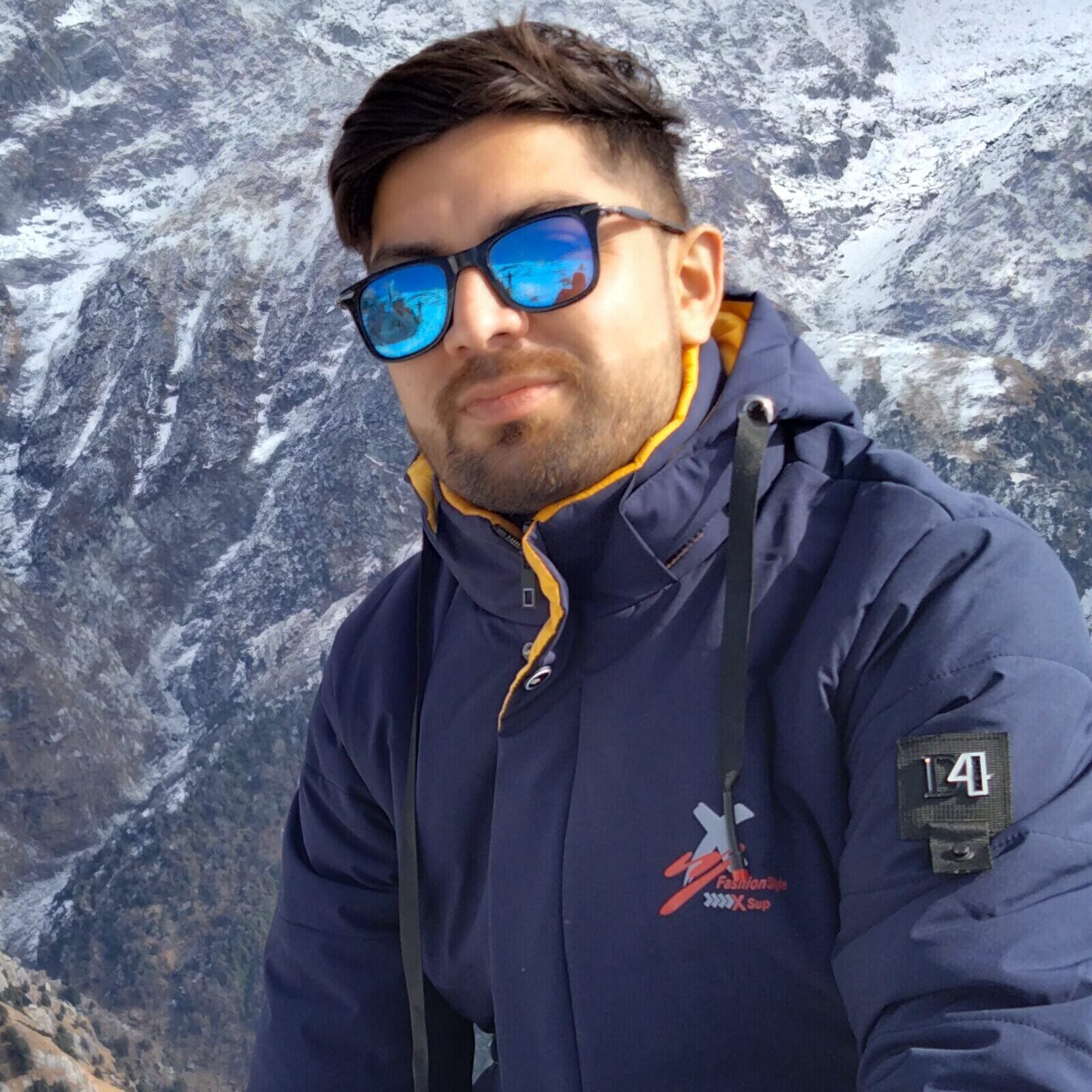
Parvesh Sandila is a passionate web and Mobile app developer from Jalandhar, Punjab, who has over six years of experience. Holding a Master’s degree in Computer Applications (2017), he has also mentored over 100 students in coding. In 2019, Parvesh founded Owlbuddy.com, a platform that provides free, high-quality programming tutorials in languages like Java, Python, Kotlin, PHP, and Android. His mission is to make tech education accessible to all aspiring developers.​