In this tutorial, we will learn how to use SeekBar in Android. The Seekbar is a child class of AbsSeekBar class. Seekbar is kind of progress bar and Seekbar allow users to move thumb of Seekbar from left or right. For example, in media players, you can move the song forward or backwards by moving a Seekbar. Here we will learn how to add SeekBar in the XML layout file and how to get change listener of SeekBar in android Java file.
Page Contents
XML Code:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:padding="16dp"
android:orientation="vertical"
android:gravity="center"
tools:context=".MainActivity">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="0"
android:layout_marginBottom="15dp"
android:id="@+id/textView"
android:textSize="18sp"/>
<SeekBar
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/seekBar"/>
</LinearLayout>
Output:
After creating this XML layout file we will move to our Java file In this file we will use seekbar change listener to track the change in seekbar.
Java Code:
public class MainActivity extends AppCompatActivity {
SeekBar seekBar;
TextView textView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
seekBar=(SeekBar)findViewById(R.id.seekBar);
textView=(TextView)findViewById(R.id.textView);
textView.setTextColor(Color.BLACK);
seekBar.setOnSeekBarChangeListener(new SeekBar.OnSeekBarChangeListener() {
@Override
public void onProgressChanged(SeekBar seekBar, int progress, boolean fromUser) {
textView.setText(progress+"");
}
@Override
public void onStartTrackingTouch(SeekBar seekBar) {
textView.setTextColor(Color.GREEN);
}
@Override
public void onStopTrackingTouch(SeekBar seekBar) {
textView.setTextColor(Color.BLACK);
}
});
}
}
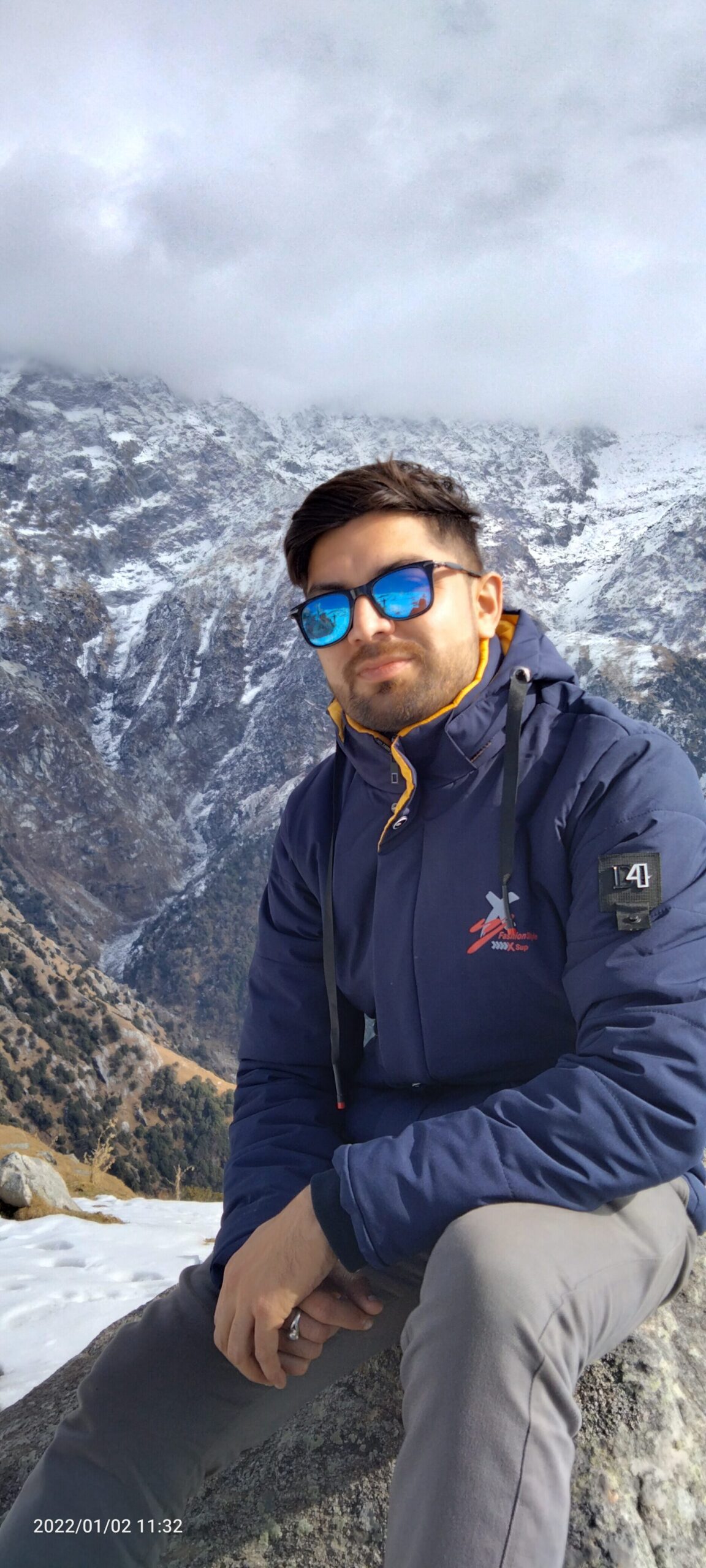
Parvesh Sandila is a passionate web and Mobile app developer from Jalandhar, Punjab, who has over six years of experience. Holding a Master’s degree in Computer Applications (2017), he has also mentored over 100 students in coding. In 2019, Parvesh founded Owlbuddy.com, a platform that provides free, high-quality programming tutorials in languages like Java, Python, Kotlin, PHP, and Android. His mission is to make tech education accessible to all aspiring developers.​