In this tutorial, we will learn about the final keyword in Java. The main motive of the final keyword is to restrict the user and final keyword can be used to create three kinds of restriction. These are:
Page Contents
Final keyword in Java with variable:
The final keyword is to make the variable final(constant). Once you declared a variable with the final keyword you can not change the value of this final variable throughout the program.
Example Program:
class Math{
final static double pi=3.14;
public static void main(String args[]){
System.out.println("Value of Pi is "+pi);
//pi=2.5; if you will try to assign value compiler will show an error
}
}
Final keyword in Java with Method:
When we make a method final in class it means we cannot override this method in child class.
Example Program:
class Math{
final static double pi=3.14;
final public void squareRoot(int num){
System.out.println("SQ ans is "+(num*num));
}
}
public class Calculator extends Math{
//if we try to override this function it will show an error
/*public void squareRoot(int num){
System.out.println("SQ ans is "+(num*num));
}*/
public static void main(String args[]){
Calculator cal=new Calculator();
cal.squareRoot(5);
}
}
Final keyword with Class:
When we use final keyword with a class, it means we can not inherit this class in any other class. The following code will show an error.
final class Math{
final static double pi=3.14;
public void squareRoot(int num){
System.out.println("SQ ans is "+(num*num));
}
}
public class Calculator extends Math{
public static void main(String args[]){
Calculator cal=new Calculator();
cal.squareRoot(5);
}
}
Have you ever tried to inherit String class in your class. You can not inherit String class because it is a final class. Click here to check.
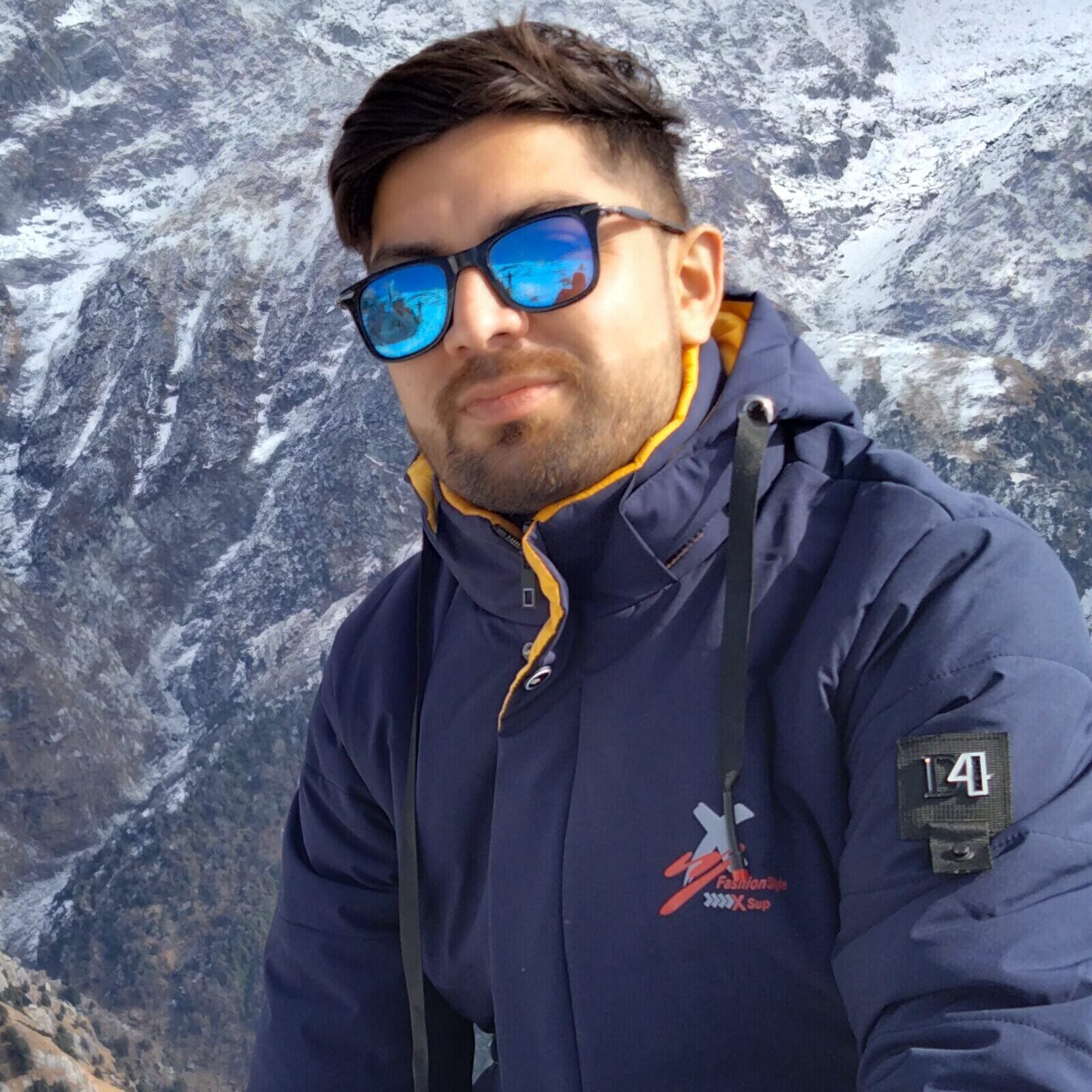
Parvesh Sandila is a passionate web and Mobile app developer from Jalandhar, Punjab, who has over six years of experience. Holding a Master’s degree in Computer Applications (2017), he has also mentored over 100 students in coding. In 2019, Parvesh founded Owlbuddy.com, a platform that provides free, high-quality programming tutorials in languages like Java, Python, Kotlin, PHP, and Android. His mission is to make tech education accessible to all aspiring developers.​