In this tutorial, we would learn about Button in Android. The button is one of the basic widgets which we normally use in each Android app. For example, in the last tutorial, we learned about EditText and in the example of EditText, we added a button in the Signup form. So same like that we can use the button for a different purpose in our Android App. We can use Button in Login form Button in the Signup form. In this tutorial, we will learn how to add button widget in our XML Layout file and how to write working of that particular button in our Java file. Firstly we would start with our XML Layout file.
Page Contents
XML Code:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center"
android:padding="16dp"
android:orientation="vertical"
tools:context=".ButtonExample">
<EditText
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="Enter Your Name"
android:inputType="text"
android:id="@+id/editText"
android:layout_marginBottom="10dp"
/>
<Button
android:layout_width="250dp"
android:layout_height="wrap_content"
android:layout_marginBottom="10dp"
android:textColor="@color/colorWhite"
android:background="@color/colorPrimary"
android:id="@+id/button"
android:text="Create my Account"/>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="18sp"
android:text="Text View"
android:textColor="@color/colorPrimary"
android:id="@+id/textView"/>
</LinearLayout>
Output of This XML layout file will look like this..
In this example you can see we added an EditText, a Button and a TextView and our purpose is to Enter a Name in EditText and to show that name in our TextView on click of our Button.
Java Code:
public class ButtonExample extends AppCompatActivity {
TextView textView;
Button button;
EditText editText;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
textView=(TextView)findViewById(R.id.textView);
button=(Button)findViewById(R.id.button);
editText=(EditText)findViewById(R.id.editText);
button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String name=editText.getText().toString();
textView.setText("Hello "+name);
}
});
}
}
Here the output of when will press Button after Entering a name in EditText
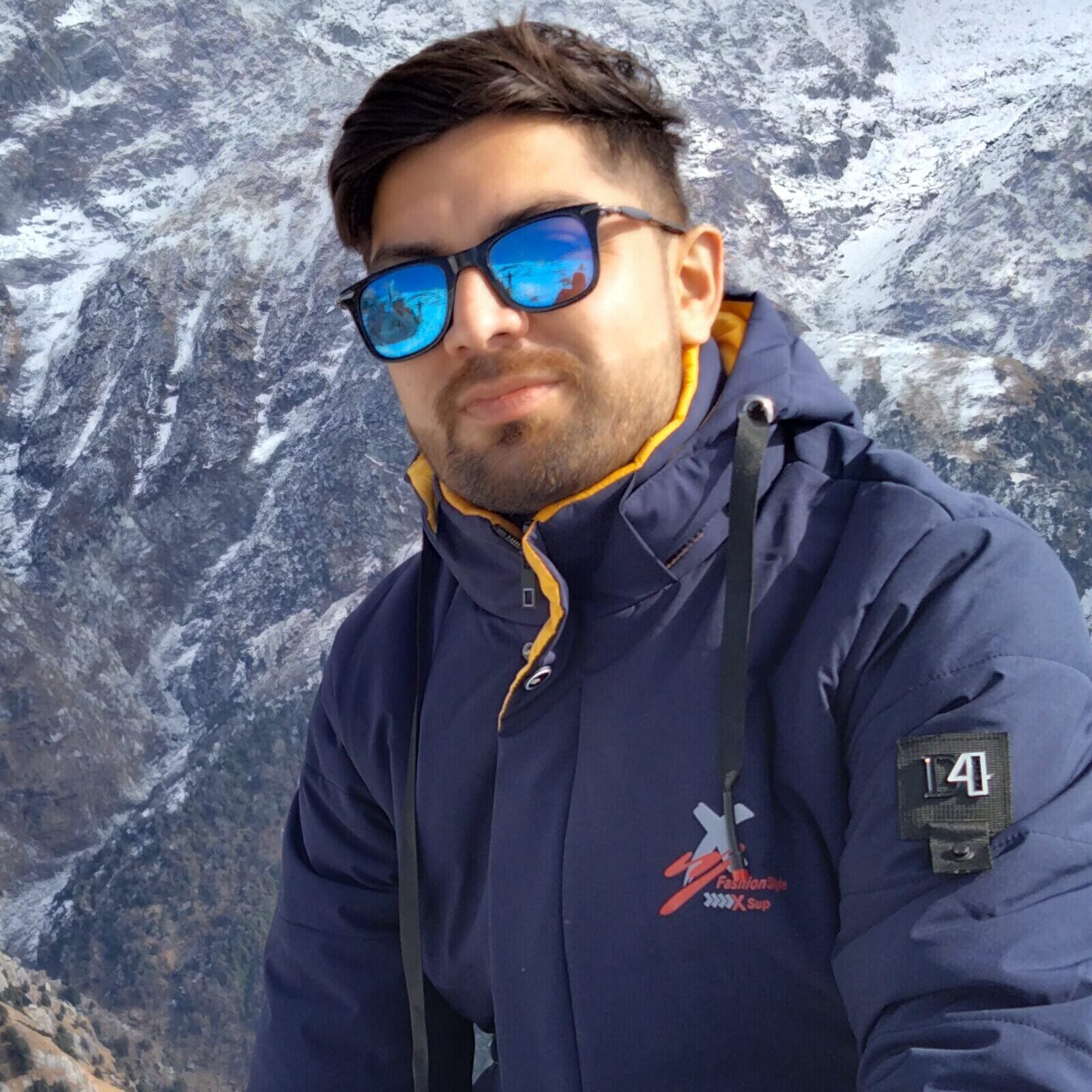
Parvesh Sandila is a passionate web and Mobile app developer from Jalandhar, Punjab, who has over six years of experience. Holding a Master’s degree in Computer Applications (2017), he has also mentored over 100 students in coding. In 2019, Parvesh founded Owlbuddy.com, a platform that provides free, high-quality programming tutorials in languages like Java, Python, Kotlin, PHP, and Android. His mission is to make tech education accessible to all aspiring developers.​