In this tutorial, we will learn about Python Tkinter MessageBox. Tkinter MessageBox widget is used to show a message box in the application. This widget comes with the number of functions to show perfect message according to the requirements of the application.
Page Contents
Available Functions in MessageBox:
- showinfo()
- showwarning()
- showerror()
- askquestion()
- askokcancel()
- askyesno()
- askretrycancel()
Syntax to add Python Tkinter MessageBox:
messagebox.function_name(title, message, [options])
- function_name: Here we have to write an appropriate function name according to the requirements of the application.
- title: This option to write the title for the MessageBox.
- message: This option to write the message in MessageBox.
- options: Here we can write various options to configure the MessageBbox.
Now we will check each function of MessageBox one by one.
showinfo():
The showinfo() method we can use where we want to show some information to user.
from tkinter import *
from tkinter import messagebox
mainWindow = Tk()
mainWindow.geometry("300x200")
messagebox.showinfo("Important","Here is an important info")
mainWindow.mainloop()
showwarning():
This function is used to show a warning message to the user.
from tkinter import *
from tkinter import messagebox
mainWindow = Tk()
mainWindow.geometry("300x200")
messagebox.showwarning("Warning","Ohh Warning message here")
mainWindow.mainloop()
showerror():
This function is used to show an error message to the user.
from tkinter import *
from tkinter import messagebox
mainWindow = Tk()
mainWindow.geometry("300x200")
messagebox.showerror("Error","Ohh Error message here")
mainWindow.mainloop()
askquestion():
This function is used to ask some question to user and user can reply to this question in the form of yes or no.
from tkinter import *
from tkinter import messagebox
mainWindow = Tk()
mainWindow.geometry("300x200")
messagebox.askquestion("Exit","Do you really want to exit?")
mainWindow.mainloop()
askokcancel():
This function is used when we need to get some kind of confirmation from the user. In this kind of message box user can click on “ok” or “cancel”.
from tkinter import *
from tkinter import messagebox
mainWindow = Tk()
mainWindow.geometry("300x200")
messagebox.askokcancel("Exit","Exit without save?")
mainWindow.mainloop()
askyesno():
This askyesno() function is used to ask a question to the user. For which the user can reply by clicking on “yes” or “no” button.
from tkinter import *
from tkinter import messagebox
mainWindow = Tk()
mainWindow.geometry("300x200")
messagebox.askyesno("Apply","Apply Changes?")
mainWindow.mainloop()
askretrycancel():
This function is use to show retry message to user to ask whether user want retry a particular task.
from tkinter import *
from tkinter import messagebox
mainWindow = Tk()
mainWindow.geometry("300x200")
messagebox.askretrycancel("Internet Error","Internet Connection interrupted.")
mainWindow.mainloop()
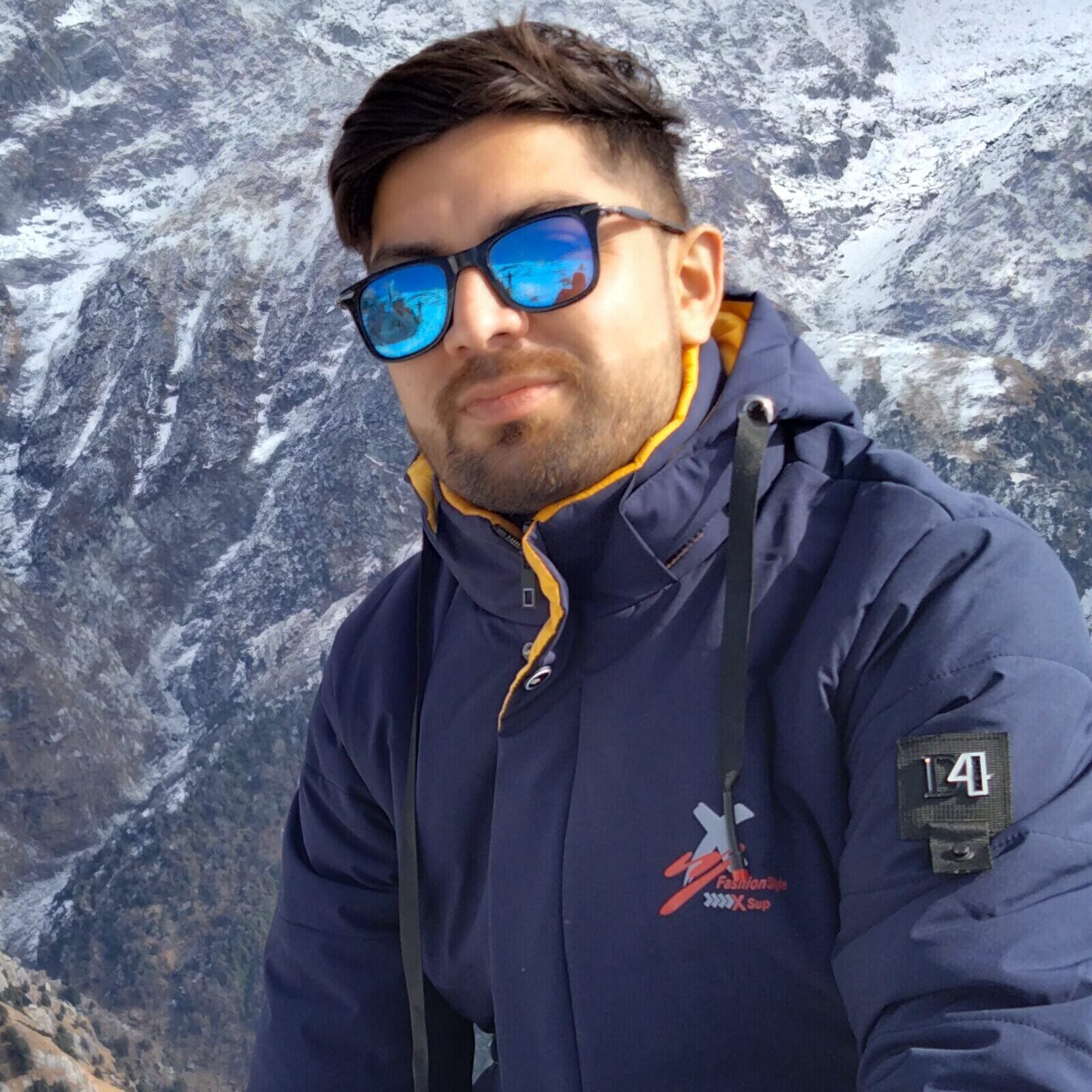
Parvesh Sandila is a passionate web and Mobile app developer from Jalandhar, Punjab, who has over six years of experience. Holding a Master’s degree in Computer Applications (2017), he has also mentored over 100 students in coding. In 2019, Parvesh founded Owlbuddy.com, a platform that provides free, high-quality programming tutorials in languages like Java, Python, Kotlin, PHP, and Android. His mission is to make tech education accessible to all aspiring developers.​