In this tutorial, we will learn about Python Tkinter Menu. Tkinter Menu helps us to add a menu in the Python application. we can create three kinds of the menu using Menu widget which is: pop-up, top-level and pull-down.
Page Contents
Syntax to add Python Tkinter Menu:
w = Menu (master, options)
- master: This represents the parent window.
- options: Here is the list of most commonly used options for this widget.
Example Program of Python Tkinter Menu:
from tkinter import *
mainWindow = Tk()
mainWindow.geometry("300x150")
menubar = Menu(mainWindow)
filemenu = Menu(menubar, tearoff=0)
filemenu.add_command(label="New")
filemenu.add_command(label="Open")
filemenu.add_command(label="Save")
filemenu.add_command(label="Save as")
menubar.add_cascade(label="File", menu=filemenu)
editmenu = Menu(menubar, tearoff=0)
editmenu.add_command(label="Undo")
editmenu.add_command(label="Cut")
editmenu.add_command(label="Copy")
editmenu.add_command(label="Paste")
editmenu.add_command(label="Delete")
editmenu.add_command(label="Select All")
menubar.add_cascade(label="Edit", menu=editmenu)
mainWindow.config(menu=menubar)
mainWindow.mainloop()
Various possible options in Python Tkinter Menu:
Option | Description |
---|---|
activebackground | This option is used to set background color of widget under focus. |
activeborderwidth | This option is used to set width of border when widget under mouse. By default it is 1px. |
activeforeground | This option is used to set foreground color of widget under focus. |
bg | This option helps us to set normal background color of widget. |
bd | This option helps us to set the border size around widget. |
cursor | This option helps us to set the style of cursor like an arrow, dot etc. |
disabledforeground | This option is used to disable text color of widget. |
font | This option is used to set font type in widget. |
fg | This option helps us to set normal foreground colour of widget. |
relief | This helps us to set the style of the border by which is default Flat. |
selectcolor | This option is used to set color of selected RadioButton or CheckBox. |
title | This option is used to set the title of the window. |
Python Tkinter Menu Methods:
After learning about various available options in Python Tkinter Menu. Its time to check out some available methods for Tkinter Menu widget.
METHOD | DESCRIPTION |
---|---|
add_command(options) | This method is used to add menu items in the menu widget. |
add_radiobutton(options) | This method is used to add radio buttons in the menu widget. |
add_checkbutton(options) | This method is used to add the check button in the menu button. |
add_cascade(options) | This method is used to add one menu into another menu to create a hierarchical menu. |
add_seperator() | This method is used to create a separator line in the menu. |
add(type, options) | This method is used to add a specific menu item in the menu widget. |
delete(startindex, endindex) | This method is used to delete all the menu items from the menu which falls between specified range, |
entryconfig(index, options) | This method is used to modify(changing options) menu item at the given index. |
index(item) | This method is used to get the index number of the specified menu item. |
insert_seperator(index) | This method is used to add a separator at a specified index. |
type(index) | This method returns the type of specified index item such as “cascade”, “checkbutton”, “command”, “radiobutton”, “separator”, or “tearoff”. |
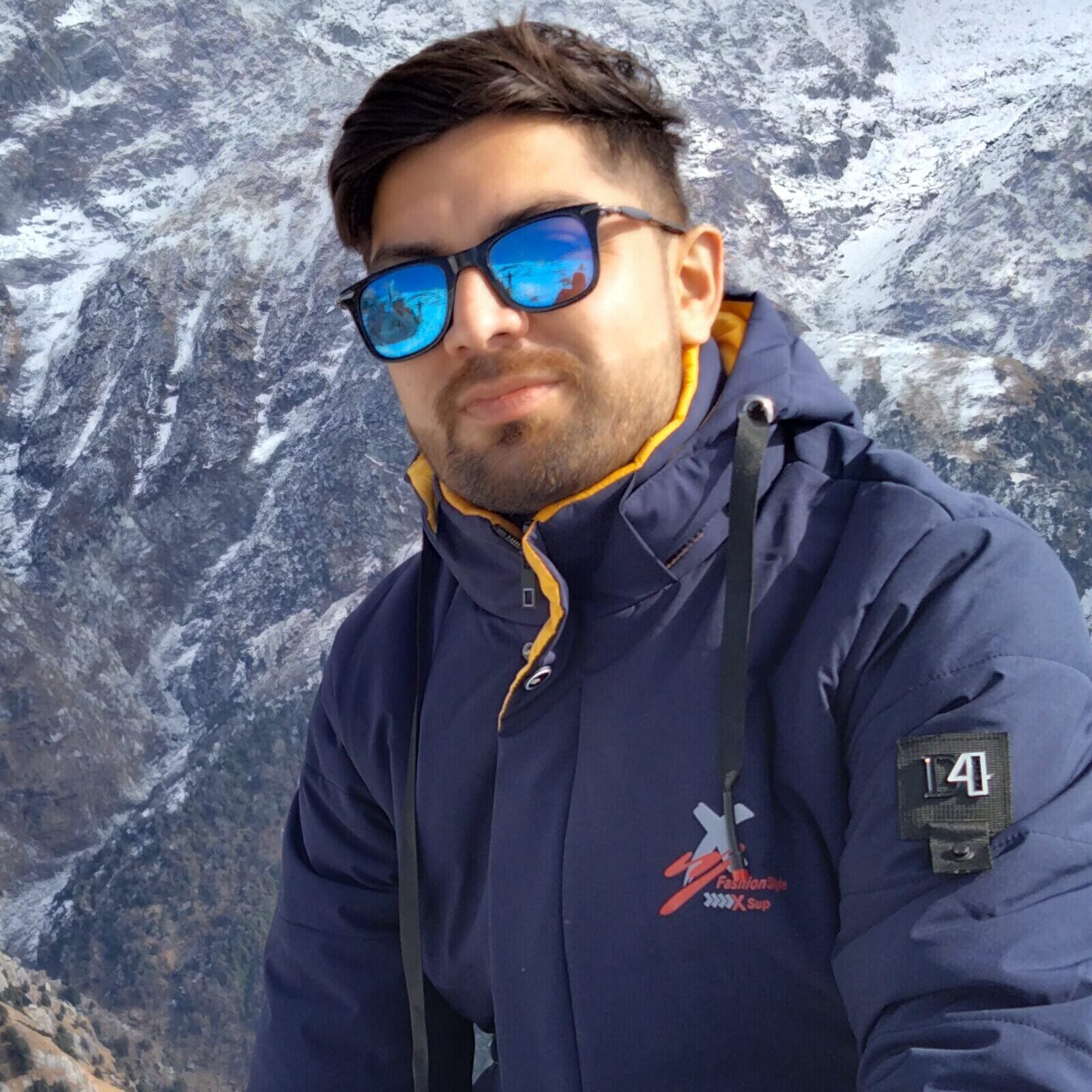
Parvesh Sandila is a passionate web and Mobile app developer from Jalandhar, Punjab, who has over six years of experience. Holding a Master’s degree in Computer Applications (2017), he has also mentored over 100 students in coding. In 2019, Parvesh founded Owlbuddy.com, a platform that provides free, high-quality programming tutorials in languages like Java, Python, Kotlin, PHP, and Android. His mission is to make tech education accessible to all aspiring developers.​