In this tutorial, we will learn about the Python Tkinter Label. Tkinter Label is used to provide a caption to widgets in Python application. Tkinter Label can be used to display normal text and images.
Page Contents
Syntax of Python Tkinter Label:
w = Label (master, options)
- master: This represents the parent window.
- options: Here is the list of most commonly used options for this widget.
Example of Python Tkinter Label:
from tkinter import *
top = Tk()
top.geometry("400x200")
username = Label(top, text = "UserName").place(x = 30,y = 50)
password = Label(top, text = "Password").place(x = 30, y = 90)
sbmitbtn = Button(top, text = "Login",activebackground = "skyblue", activeforeground = "white").place(x = 30, y = 130)
usernameE = Entry(top).place(x = 100, y = 50)
passwordE = Entry(top).place(x = 100, y = 90)
top.mainloop()
Various possible options in Tkinter Label:
Option | Description |
---|---|
anchor | This option helps us to set position of text inside the widget. By default value of anchor is CENTER. |
bg | This option helps us to set normal background color of widget. |
bitmap | This option helps us to display an image using label. You just have to set this option equal to an image object. |
bd | This option helps us to set the border size around widget. |
cursor | This option helps us to set the style of cursor like an arrow, dot etc |
font | This option helps us to set the style of font. |
fg | This option helps us to set normal foreground colour of widget. |
height | This option helps us to set height of widget. |
justify | This helps us to automatically organize the text in multiple lines. |
padx | This option helps us to set space left and right of widget. |
pady | This option helps us to set space above and below of widget. |
relief | This helps us to set the style of the border by which is default Flat. |
text | This option helps us to set string source for label. |
underline | This option helps us to underline specified letter of the text. |
width | This helps us to set the width of the widget. |
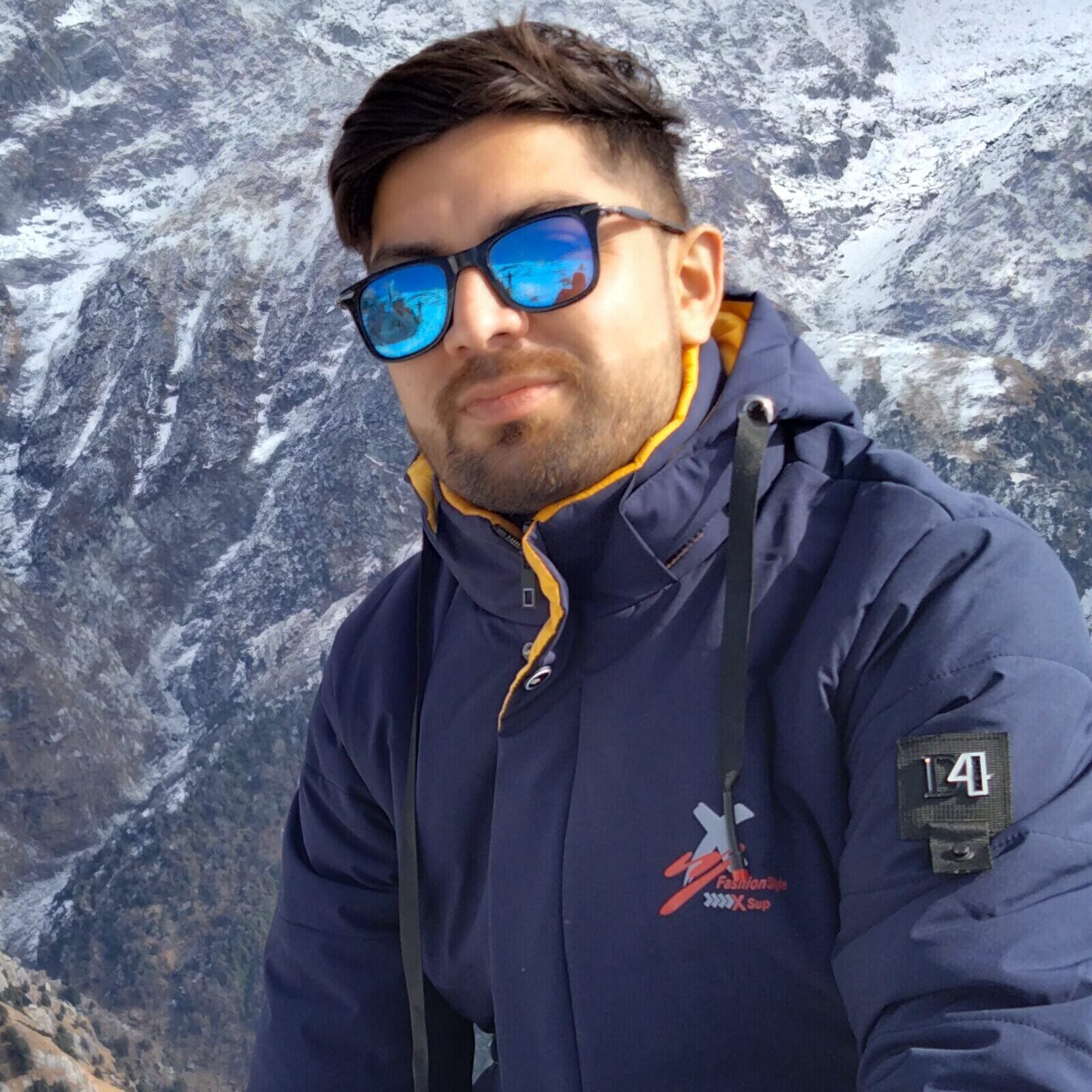
Parvesh Sandila is a passionate web and Mobile app developer from Jalandhar, Punjab, who has over six years of experience. Holding a Master’s degree in Computer Applications (2017), he has also mentored over 100 students in coding. In 2019, Parvesh founded Owlbuddy.com, a platform that provides free, high-quality programming tutorials in languages like Java, Python, Kotlin, PHP, and Android. His mission is to make tech education accessible to all aspiring developers.​