In this tutorial, we will learn about Operators in Python. Operators are special symbols which normally use to perform some special task in the program. For example, you want to add two number. Here we will use + operator to add two numbers or you want to assign value to a variable. Here we will use = assignment operator to assign value to a variable. There are so many operators available in Python and they are divided into different categories. We learn here all the categories.
Page Contents
Arithmetic operators:
Arithmetic operators are so simple. Arithmetic operators are used to performing simple mathematical computations like addition, subtraction, multiplication and division. There are total of seven operators in this category and these are as follow.
Operator | Description | Example |
---|---|---|
+ | Addition: This Operator is used to add Two numbers in Python | 10+15 |
– | Subtraction: This Operator is used to subtract the second operand from first. | 20-10 |
* | Multiply: This Operator is used to multiply two operands. | 10*2 |
/ | Division: This Operator is used to divide the first operand by second. | 10/2 |
% | Modulus: This Operator returns the remainder after dividing the first operand by the second operand. | 10%2 |
// | Floor Division: It will return non-decimal value after division e.g. result of 5//2 = 2 | 5//2 |
** | Exponent: This operator is used to the first operand raised to the power of the second operand | 10**2 |
Example of Arithmetic Operators in Python:
num1 = 20
num2 = 5
# Output: num1 + num2 = 25
print('num1 + num2 =',num1+num2)
# Output: num1 - num2 = 15
print('num1 - num2 =',num1-num2)
# Output: num1 * num2 = 100
print('num1 * num2 =',num1*num2)
# Output: num1 / num2 = 4.0
print('num1 / num2 =',num1/num2)
# Output: num1 // num2 = 4
print('num1 // num2 =',num1//num2)
# Output: num1 ** num2 = 3200000
print('num1 ** num2 =',num1**num2)
Identity Operators:
Identity Operators are used to comparing two objects. It will return true if both objects share the same memory location.
Operator | Description | Example |
---|---|---|
is | This operator will return true if both objects are on the same memory location. | x is y |
is not | This operator will return true if both objects are not on the same memory location. | x is not y |
Example of Identity operators in Python:
str1 = "Owlbuddy"
str2 = str1
print(str1 is str2)
print(str1 is not str2)
Membership Operators:
Membership operators in Python are used to check if a sequence is present in Object.
Operator | Description | Example |
---|---|---|
in | Return true if the given sequence is present in the given object. | x in y |
not in | Returns true if a given sequence is not present in Object. | x not in y |
Example of Membership operators in Python:
names = ["Ravi", "Gurpreet"]
print("Ravi" in names)
print("Hardeep" not in names)
Comparison operators:
Comparison operators are used to comparing two values in Python and the result of this comparison will come like true or false. Here are some operators which come in this category.
Operator | Description | Example |
---|---|---|
> | Greater than: This Operator will return true if left side operand will greater than operand at the right side. | 20>10 |
< | Less Than: This Operator will return true if left side operand will be smaller then operand at the right side. | 10>20 |
>= | Greater than equal to: This operator will return to if operand at the left side will greater or equal to operand at the right side. | 20>=10 |
<= | Less than equal to: This operator will return to if operand at the left side will small or equal to operand at the right side. | 10<=20 |
== | Equal to: This Operator will return true if operands of both sides will be equal. | 10==10 |
!= | Not Equal: This Operator will return true if operands at both sides will not equal. | 10!=20 |
Example of Comparison operators in Python:
num1 = 20
num2 = 25
# Output: num1 > num2 is False
print('num1 > num2 is',num1 > num2)
# Output: num1 < num2 is True
print('num1 < num2 is',num1 < num2)
# Output: num1 == num2 is False
print('num1 == num2 is',num1 == num2)
# Output: num1 != num2 is True
print('num1 != num2 is',num1 != num2)
# Output: num1 >= num2 is False
print('num1 >= num2 is',num1 >= num2)
# Output: num1 <= num2 is True
print('num1 <= num2 is',num1 <= num2)
Logical operators in Python:
Logical operators are used to making comparison between two expressions. There are three logical operators in Python.
Operator | Description | Example |
---|---|---|
and | Logical and: This Operator returns true if both operands will be true | x and y |
or | Logical or: This Operator returns true if any one or both operand will be true | x or y |
not | Logical not: This Operator return compliment result of the given operand | x or y |
Example of Logical operators in Python:
x = True
y = False
# Output: Result of x and y is False
print('Result of x and y is',x and y)
# Output: Result of x or y is True
print('Result of x or y is',x or y)
# Output:Result of not x is False
print('Result of not x is',not x)
Assignment operators in Python:
Assignment operators are used to assigning value to a variable in Python. There is a rich collection of assignment operators in Python.
Operator | Description | Example |
---|---|---|
= | Equal to an operator to assign value to a variable. | x = 5 |
+= | Plus Equal to an operator to assign value to the variable after adding given value in a previous value of a variable. | x += 5 |
-= | Minus Equal to an operator to assign value to the variable after subtracting given value from the previous value of a variable. | x -= 5 |
*= | Multiply Equal to an operator to assign value to the variable after Multiplying given value with the previous value of the variable. | x *= 5 |
/= | Divide Equal to an operator to assign value to the variable after Dividing given value from the previous value of the variable. | x /= 5 |
%= | Modulus Equal to an operator to assign Remainder to the variable after Dividing given value from the previous value of the variable. | x %= 5 |
//= | Divide(floor) Equal to an operator to assign a value(floor) to the variable after Dividing given value from the previous value of the variable. | x //= 5 |
**= | Exponent Equal to an operator to assign exponent(square) to the variable after calculating. | x **= 5 |
&= | Bitwise AND Equal to an operator to assign value to the variable after performing bitwise operator. | x &= 5 |
|= | Bitwise OR Equal to an operator to assign value to the variable after performing Bitwise OR operator. | x |= 5 |
^= | Bitwise XOR Equal to an operator to assign value to the variable after performing XOR operator. | x ^= 5 |
>>= | Bitwise right shift Equal to operator to assign value to the variable after performing bitwise right shift. | x >>= 5 |
<<= | Bitwise Left shift Equal to an operator to assign value to the variable after performing Bitwise left shift. | x <<= 5 |
Example of Assignment Operators in Python:
num = 3
print("Value of num= "+num)
num+=10
print("Value of num= "+num)
num-=5
print("Value of num= "+num)
num*=2
print("Value of num= "+num)
num/=2
print("Value of num= "+num)
num%=2
print("Value of num= "+num)
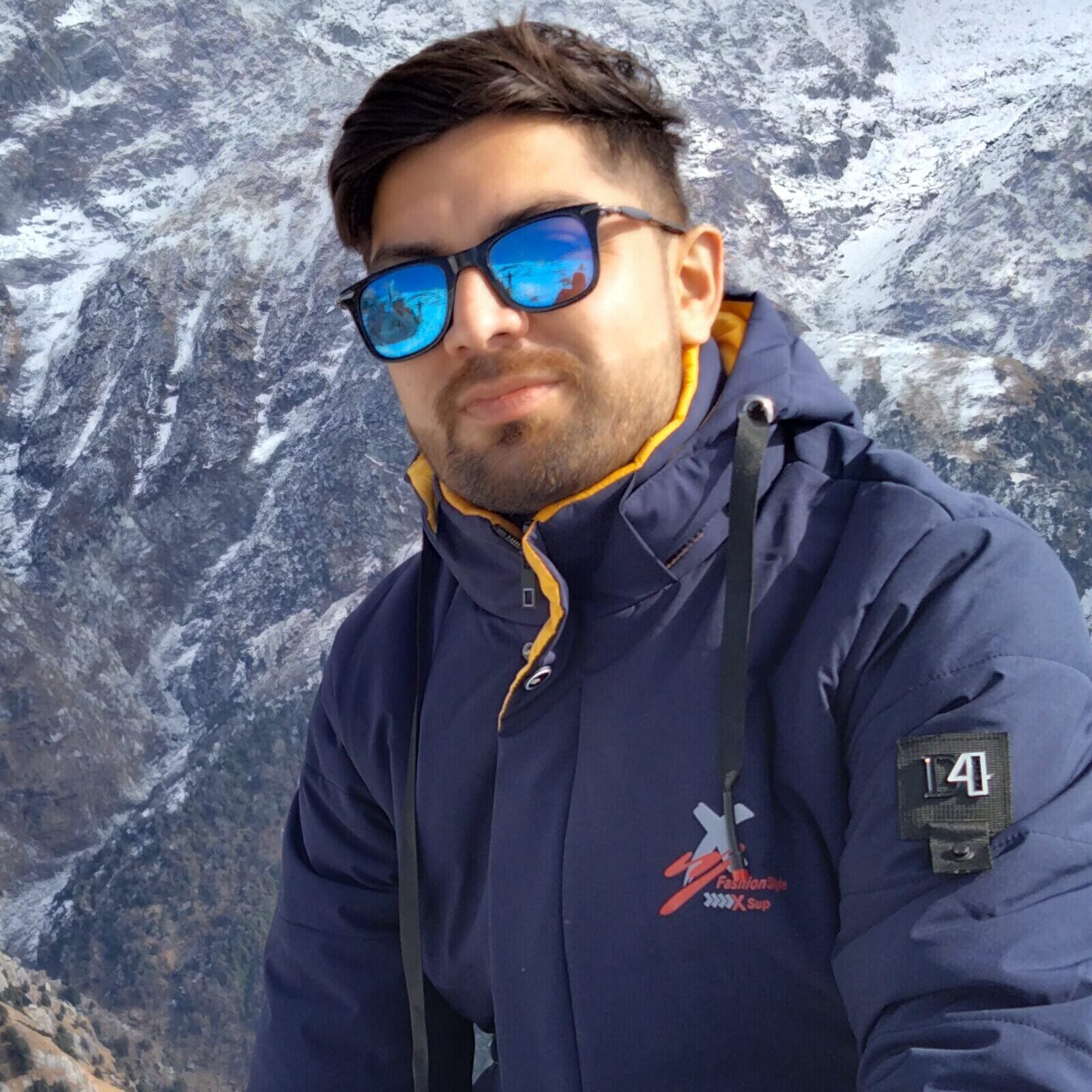
Parvesh Sandila is a passionate web and Mobile app developer from Jalandhar, Punjab, who has over six years of experience. Holding a Master’s degree in Computer Applications (2017), he has also mentored over 100 students in coding. In 2019, Parvesh founded Owlbuddy.com, a platform that provides free, high-quality programming tutorials in languages like Java, Python, Kotlin, PHP, and Android. His mission is to make tech education accessible to all aspiring developers.​