In this tutorial, we will learn about Exception Handling in Kotlin. According to the dictionary, the word exception means abnormal and Here Exception handling means to solve abnormal situations which occur while the execution of code.
Page Contents
During the execution of the program due to several reasons such as wrong input, or some programming mistakes, the program stops working and throw an error on screen which is known exception. Kotlin has a concept called Exception Handling to handle these exceptions.
Example Program:
fun main(args : Array<String>){
println("Ans of 10/2 ="+(10/2))
println("Ans of 12/3 ="+(12/3))
println("Ans of 5/0 ="+(5/0)) // this will throws ArithmeticException
println("Ans of 24/6 ="+(24/6))
println("Ans of 8/2 ="+(8/2))
}
Output:
Ans of 10/2 =5
Ans of 12/3 =4
Exception in thread "main" java.lang.ArithmeticException: / by zero
As in the above example, you can see our code was working perfectly till the 4th line. But when it reached to 5th line and we tried to divide 5 by 0. We got an exception(In Kotlin / by Zero is an Exception) and our program stropped to working immediately as two can see there were two more lines(line number 6th and 7th) which were not executed because of Exception.
Types of Exception:
Kotlin has two kinds of exception which can occur in Kotlin programs. Types of Exception are as follow:
- Checked Exception
- Unchecked Exception
Checked Exception:
The checked exceptions are those exceptions which can be caught on compilation time by the compiler. These kinds of exceptions can not be ignored by the compiler. The programmer has to solve these exceptions first to run the program(without solving these checked exceptions program would not compile).
List of Checked Exceptions in Kotlin:
- ClassNotFoundException
- NoSuchFieldException
- NoSuchMethodException
- InterruptedException
- IOException
- InvalidClassException
- SQLException
- any many more…
Unchecked Exception:
Unchecked Exceptions are those exceptions which can not be caught at the time of compilation of code. These exceptions only occur while the execution of code and stops the code from working.
List of Unhecked Exceptions in Kotlin:
- ArithmeticException
- ArrayIndexOutOfBoundsException
- EnumConstantNotPresentException
- NegativeArraySizeException
- NumberFormatException
- NullPointerException
- IllegalStateException
- UnsupportedOperationException
- and many more..
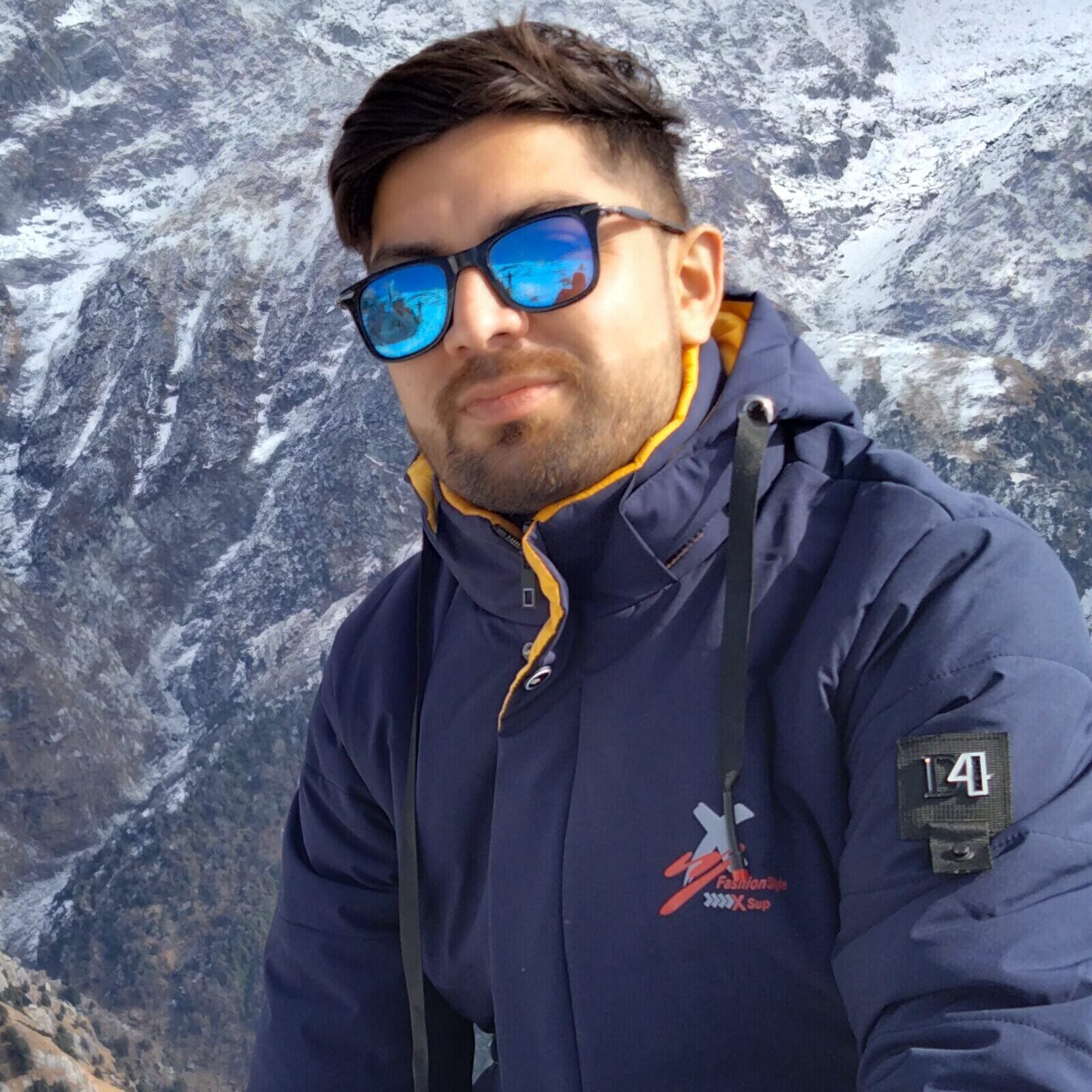
Parvesh Sandila is a passionate web and Mobile app developer from Jalandhar, Punjab, who has over six years of experience. Holding a Master’s degree in Computer Applications (2017), he has also mentored over 100 students in coding. In 2019, Parvesh founded Owlbuddy.com, a platform that provides free, high-quality programming tutorials in languages like Java, Python, Kotlin, PHP, and Android. His mission is to make tech education accessible to all aspiring developers.​