In this tutorial, we will learn about Operators in C programming language. Operators are special symbols that we use to perform special operations such as the addition of two numbers(variables).
Page Contents
C provides us with a wide range of operators which helps us to perform special operations. In C, all the operators are divided into different categories
- Arithmetic Operators
- Relational Operators
- Logical Operators
- Bitwise Operators
- Assignment Operators
- Misc Operators
Arithmetic Operators:
Arithmetic operators in C are used to perform simple mathematical operations like Addition, subtraction, multiplication, division, etc.
Operator | Description | Example |
---|---|---|
+ | This operator is used to add to operands. | 10+10=20 |
− | This operator is used to subtract the right-hand side operand from the left-hand side operand. | 20-5=15 |
* | This operator is used to multiply both operands. | 20*2=40 |
/ | This operator is used to divide the left-hand side operand with the right-hand side operand. | 15/3=5 |
% | This operator is used to get remainder after performing division. | 15/2=1 |
Example Program:
#include <stdio.h>
int main()
{
int a=21;
int b=5;
printf("Addition = %d\n",a+b);
printf("Subtraction = %d\n",a-b);
printf("Multiplication = %d\n",a*b);
printf("Division = %d\n",a/b);
printf("Modulous = %d\n",a%b);
return 0;
}
Output:
Addition = 26
Subtraction = 16
Multiplication = 105
Division = 4
Modulous = 1
Relational Operators:
Relational operators are used to checking the relation between two operands such as greater than, less than, equals to, etc. If the relation between two operands is true then the result of the relational expression will be 1 if the relation is false then the result of the relational expression will 0
.
Operator | Description | Example |
---|---|---|
> | This operator will return true if the left side operand is greater than the right side operand. | 10>5=1 |
>= | This operator will return true if the left side operand is greater than or equal to the right side operand. | 10>=15=0 |
< | This operator will return true if the left side operand is less than the right side operand. | 5<2=0 |
<= | This operator will return true if the left side operand is less than or equal to the right side operand. | 5<=10=1 |
== | This operator will return true if operators at both sides are equal. | 20==10=0 |
!= | This operator will return true if operators at both sides are not equal. | 20!=10=1 |
Example Program:
#include <stdio.h>
int main()
{
printf("Greater than = %d\n",10>5);
printf("Greater than equals to= %d\n",10>=15);
printf("Less than = %d\n",5<2);
printf("Less than equals to = %d\n",5<=10);
printf("Equals to = %d\n",20==10);
printf("Not Equal to = %d\n",20!=10);
return 0;
}
Output:
Greater than = 1
Greater than equals to= 0
Less than = 0
Less than equals to = 1
Equals to = 0
Not Equal to = 1
Logical Operators:
Generally, logical operators are used with relation expressions but we can also use logical operators with constants. If the result of the logical operator is true then 1
will return otherwise 0
will return.
Operator | Description | Example |
---|---|---|
&& | This operator is called Logical AND, It returns true if operands on both sides are true. | 1&&1=1 |
|| | This operator is called Logical OR, It returns true if any of the two operands is true. | 1||0=1 |
! | This operator is called Logical NOT, It returns true if an operand is false and false if an operand is true. | !1=0 |
Example Program:
#include <stdio.h>
int main()
{
printf("Result of 0&&0 = %d\n",0&&0);
printf("Result of 1&&0 = %d\n",1&&0);
printf("Result of 0&&1 = %d\n",0&&1);
printf("Result of 1&&1 = %d\n",1&&1);
printf("Result of 0||0 = %d\n",0||0);
printf("Result of 1||0 = %d\n",1||0);
printf("Result of 0||1 = %d\n",0||1);
printf("Result of 1||1 = %d\n",1||1);
printf("Result of !1 = %d\n",!1);
printf("Result of !0 = %d\n",!0);
return 0;
}
Output:
Result of 0&&0 = 0
Result of 1&&0 = 0
Result of 0&&1 = 0
Result of 1&&1 = 1
Result of 0||0 = 0
Result of 1||0 = 1
Result of 0||1 = 1
Result of 1||1 = 1
Result of !1 = 0
Result of !0 = 1
Bitwise Operators:
The Bitwise operators are used to perform operations on the bit level of operands. There is a total of six bitwise operators available in the C language.
Operator | Description | Example |
---|---|---|
& | This operator is called Bitwise AND. The output of this operator is 1 if the corresponding bits of two operands is 1 | 10&5=0 |
| | This operator is called Bitwise OR. The output of this operator is 1 if at least one corresponding bit of two operands is 1 | 10|5=15 |
^ | This operator is called Bitwise XOR. The result of this operator is 1 if the corresponding bits of two operands are opposite | 10^5=15 |
~ | This operator is called Bitwise Complement and it is a unary operator. It changes bits from 1 to 0 and from 0 to 1 | ~10=11 |
<< | This operator is called Bitwise Left Shift. This operator shifts all bits towards the right by a certain number of specified bits | 10<<2=40 |
>> | This operator is called Bitwise Right Shift. This operator shifts all bits towards left by a certain number of specified bits | 10>> 2=2 |
Example Program:
#include <stdio.h>
int main()
{
printf("Result of 10&5 = %d\n",10&5);
printf("Result of 10|5 = %d\n",10|5);
printf("Result of 10^5 = %d\n",10^5);
printf("Result of ~10 = %d\n",~10);
printf("Result of 10<<2 = %d\n",10<<2);
printf("Result of 10>>2 = %d\n",10>>2);
return 0;
}
Output:
Result of 10&5 = 0
Result of 10|5 = 15
Result of 10^5 = 15
Result of ~10 = -11
Result of 10<<2 = 40
Result of 10>>2 = 2
Assignment Operators:
Assignment operators are used to assigning some value to a variable. The most common use assignment operator is =.
Operator | Description | Example |
---|---|---|
= | This simple assignment operator is used to assign a right-hand side value to a variable on the left-hand side. | int a=0; a=10; Here variable a have value of 10. |
+= | This simple add assignment operator is used to assign value to a variable at the left-hand side after adding left and right-hand side operands. | int a=10; a+=10; Here variable a have value of 20. |
-= | This subtracts assignment operator is used to assigning value to a variable at the left-hand side after subtracting the right-hand side operand from the left-hand side operand. | int a=10; a-=2; Here variable a have value 8. |
*= | This simple multiply assignment operator is used to assign value to a variable at the left-hand side after multiplying left and right-hand side operands. | int a=10; a*=2; Here a have value of 16. |
/= | This subtracts assignment operator is used to assigning value to a variable at the left-hand side after dividing the left-hand side operand with the right-hand side operand. | int a=10; a/=2; Here variable a have value 5. |
%= | This subtracts assignment operator is used to assigning modulus to a variable at the left-hand side after dividing the left-hand side operand with the right-hand side operand. | int a=11; a%=2; Here variable a have value 1. |
<<= | This Bitwise Left Shift Assignment operator is used to assign value to the variable after performing bitwise lift shift operation. | int a=10; a<<=2; Here variable a have value of 40. |
>>= | This Bitwise Right Shift Assignment operator is used to assign value to the variable after performing bitwise right shift operation. | int a=10; a>>=2; Here variable a have value 2. |
&= | This Bitwise AND Assignment operator is used to assign value to the variable after performing bitwise and operation. | int a=10; a&=2; Here variable a have value 2. |
^= | This Bitwise XOR Assignment operator is used to assign value to the variable after performing bitwise xor operation. | int a=10; a^=2; Here variable a have value 8. |
|= | This Bitwise NOT Assignment operator is used to assign value to the variable after performing bitwise not operation. | int a=10; a|=2; Here variable a have value of 10. |
Example Program:
#include <stdio.h>
int main()
{
int a=0;
printf("Simple assignment = %d\n",a);
a+=10;
printf("Add Assignment = %d\n",a);
a=10;
a-=2;
printf("Subtract Assignment = %d\n",a);
a=10;
a*=2;
printf("Multiply Assignment = %d\n",a);
a=10;
a/=2;
printf("Divide Assignment = %d\n",a);
a=10;
a%=2;
printf("Modulous Assignment = %d\n",a);
a=10;
a<<=2;
printf("Bitwise Left Sift Assignment = %d\n",a);
a=10;
a>>=2;
printf("Bitwise Right Sift Assignment = %d\n",a);
a=10;
a&=2;
printf("Bitwise AND Assignment = %d\n",a);
a=10;
a|=2;
printf("Bitwise OR Assignment = %d\n",a);
a=10;
a^=2;
printf("Bitwise XOR Assignment = %d\n",a);
return 0;
}
Output:
Simple assignment = 0
Add Assignment = 10
Subtract Assignment = 8
Multiply Assignment = 20
Divide Assignment = 5
Modulous Assignment = 0
Bitwise Left Sift Assignment = 40
Bitwise Right Sift Assignment = 2
Bitwise AND Assignment = 2
Bitwise OR Assignment = 10
Bitwise XOR Assignment = 8
Misc Operators:
There are some miscellaneous operators in the C language. Check out the following list.
Operator | Description | Example |
---|---|---|
sizeof() | This operator returns the size of the given variable. | int a=10; sizeof(a) will return 4. |
& | This operator returns the address of the given variable. | &a |
* | This operator is used to create the pointer variable. | *a; |
? : | This conditional operator is used as a short form of the if-else statement. | if Condition ? run in if the condition is true : run in if the condition is false |
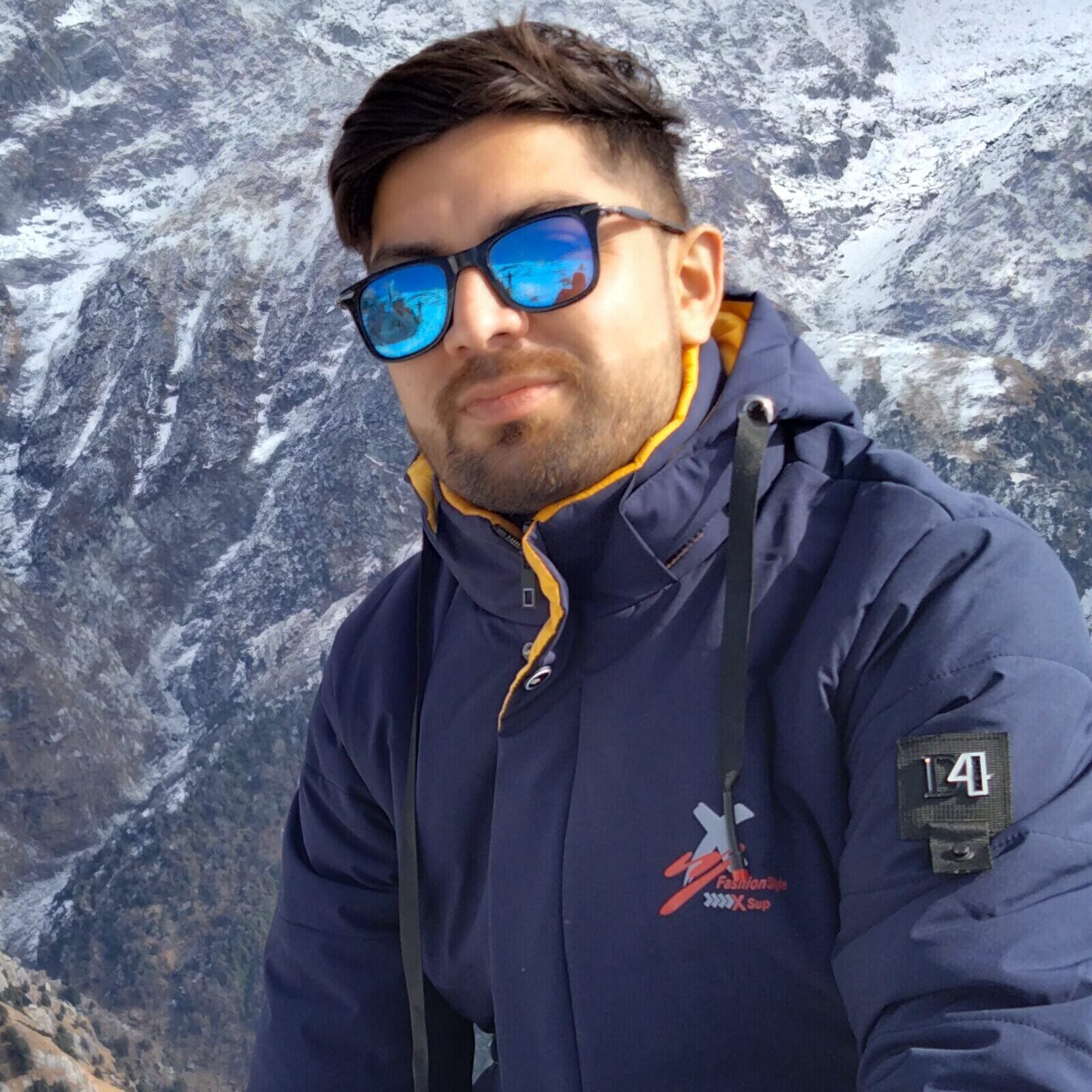
Parvesh Sandila is a passionate web and Mobile app developer from Jalandhar, Punjab, who has over six years of experience. Holding a Master’s degree in Computer Applications (2017), he has also mentored over 100 students in coding. In 2019, Parvesh founded Owlbuddy.com, a platform that provides free, high-quality programming tutorials in languages like Java, Python, Kotlin, PHP, and Android. His mission is to make tech education accessible to all aspiring developers.​