React Native has made it easy for developers to create mobile apps for both Android and iOS using JavaScript and React. Whether you’re an experienced web developer or completely new to mobile development, getting started with React Native is simple and rewarding. In this Getting Started with React Native guide, we’ll walk you through setting up your development environment, creating your first React Native app, and understanding the project structure.
Page Contents
Setting Up Your Development Environment
Before we can start building with React Native, you need to set up the required tools on your system. Follow these steps to install the necessary software for getting started with React Native:
Step 1: Install Node.js and npm
React Native requires Node.js and npm (Node package manager). Download and install the latest version of Node.js from nodejs.org.
To check if Node.js and npm are installed correctly, run the following commands in your terminal:
node -v
npm -v
You should see the versions of Node.js and npm printed to the terminal.
Step 2: Install React Native CLI or Expo CLI
There are two ways to get started with React Native:
- React Native CLI: Suitable for developers who want more control over the project setup and wish to work with native code.
- Expo CLI: A simpler tool for quickly building React Native apps without needing to configure native environments.
For this tutorial, we’ll use the Expo CLI, which is beginner-friendly.
To install Expo CLI, run:
npm install -g expo-cli
Step 3: Create Your First React Native Project
Now, let’s create our first React Native app. Run the following command to generate a new project:
npx create-expo-app MyFirstApp
cd MyFirstApp
Step 4: Running Your React Native App
You can run your app on an emulator, real device, or Expo Go app (on your phone).
To start your app, run:
npx expo start
- For Android devices: Scan the QR code in your Expo app.
- For iOS devices: Scan the QR code using your phone’s camera and open the Expo Go app.
Your first React Native app should now be running on your device!
Understanding the Project Structure
After creating your React Native project, it’s essential to understand the project structure. Here’s a quick overview of the most important files and folders:
- App.js: The entry point of your application. This is where you’ll write most of your code for now.
- assets: Contains static assets like images and fonts.
- node_modules: All project dependencies installed via npm live here.
- package.json: Contains metadata about the project, including the dependencies.
Here’s what a basic App.js
file looks like in a new React Native project:
import React from 'react';
import { Text, View } from 'react-native';
export default function App() {
return (
<View style={{ flex: 1, justifyContent: 'center', alignItems: 'center' }}>
<Text>Welcome to My First React Native App!</Text>
</View>
);
}
This code sets up a simple “Welcome” message in the centre of the screen. The View
component acts as a container, and the Text
component displays the text.
Components in React Native
React Native is all about components. Components are the building blocks of any React Native app. Here are some essential components you’ll use frequently when getting started with React Native:
- View: A container that supports layout with Flexbox.
- Text: Used for displaying text.
- Image: Renders images in your app.
- Button: Displays a pressable button.
Here’s an example of using some basic components:
import React from 'react';
import { View, Text, Button, Image } from 'react-native';
export default function App() {
return (
<View style={{ flex: 1, justifyContent: 'center', alignItems: 'center' }}>
<Text>Hello, React Native!</Text>
<Image
source={{ uri: 'https://reactnative.dev/img/tiny_logo.png' }}
style={{ width: 50, height: 50 }}
/>
<Button title="Click Me" onPress={() => alert('Button Pressed')} />
</View>
);
In this example, the Image
component loads a logo from a URL and the Button
component triggers an alert when pressed.
JSX in React Native
JSX (JavaScript XML) is a syntax extension for JavaScript that looks similar to HTML. It’s used in both React and React Native to define the structure of the UI. Here’s a basic example of JSX:
<View>
<Text>Hello, world!</Text>
</View>
In React Native, JSX elements like View
and Text
are translated into native components under the hood, which makes it look and behave like a native app.
Running the App on an Emulator or Device
You can test your React Native app on both physical devices and emulators:
- For Android: You can use Android Studio to create an Android Virtual Device (AVD). Once the emulator is running, you can launch your app with
npx expo start
, and the app will automatically open in the emulator. - For iOS: You’ll need Xcode installed on a Mac to run an iOS simulator. Once Xcode is set up, you can launch the iOS simulator from the Expo development tools.
Running the app on real devices is also easy using the Expo Go app. Simply scan the QR code from your terminal, and your app will open on your mobile device.
Conclusion
Congratulations! You’ve completed your first React Native app. In this Getting Started with React Native guide, we covered everything from setting up the environment to understanding the project structure and running the app on different devices. This is just the beginning, and there’s so much more to explore in React Native. In the next article, we’ll dive deeper into React Native components and how to use them to build rich user interfaces.
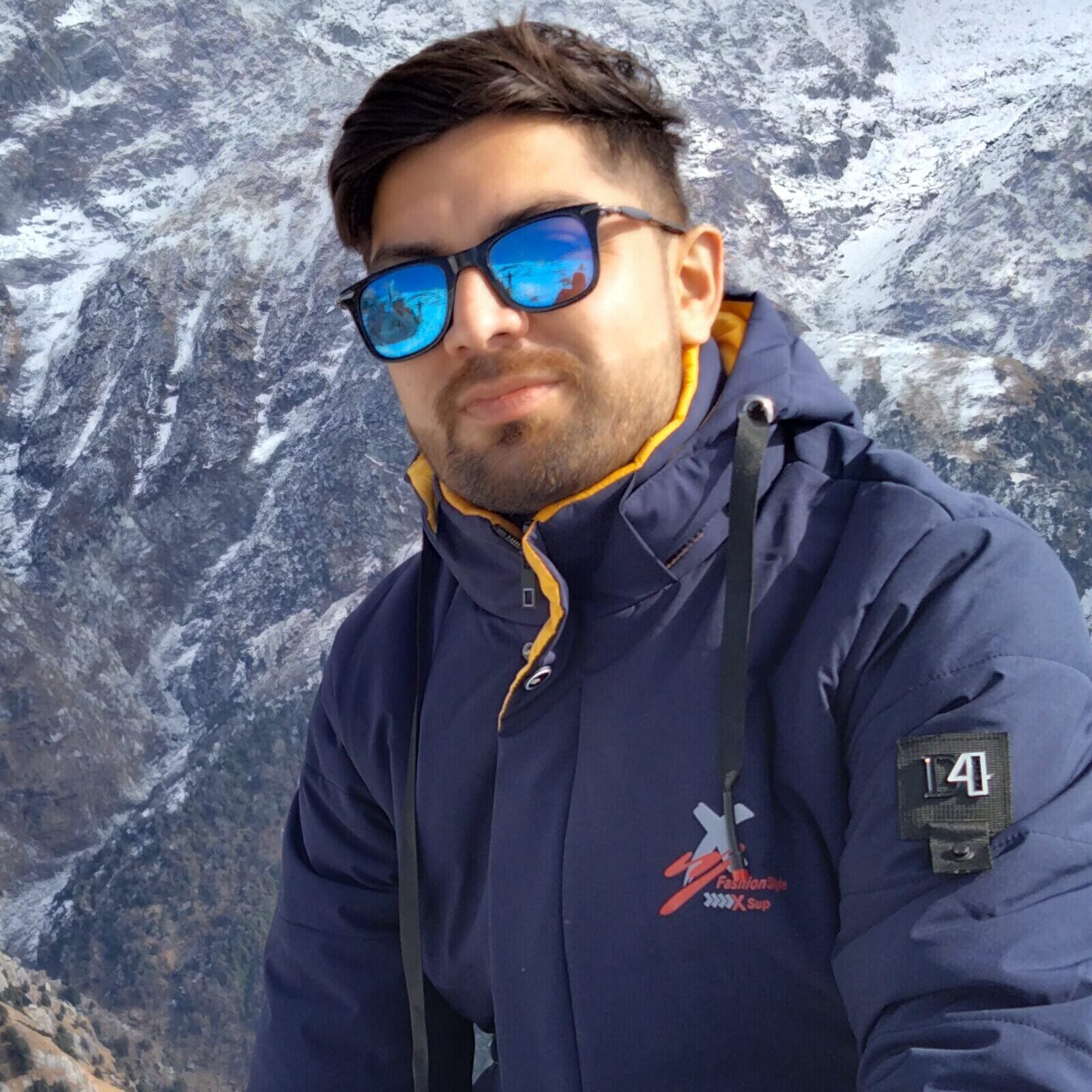
Parvesh Sandila is a passionate web and Mobile app developer from Jalandhar, Punjab, who has over six years of experience. Holding a Master’s degree in Computer Applications (2017), he has also mentored over 100 students in coding. In 2019, Parvesh founded Owlbuddy.com, a platform that provides free, high-quality programming tutorials in languages like Java, Python, Kotlin, PHP, and Android. His mission is to make tech education accessible to all aspiring developers.